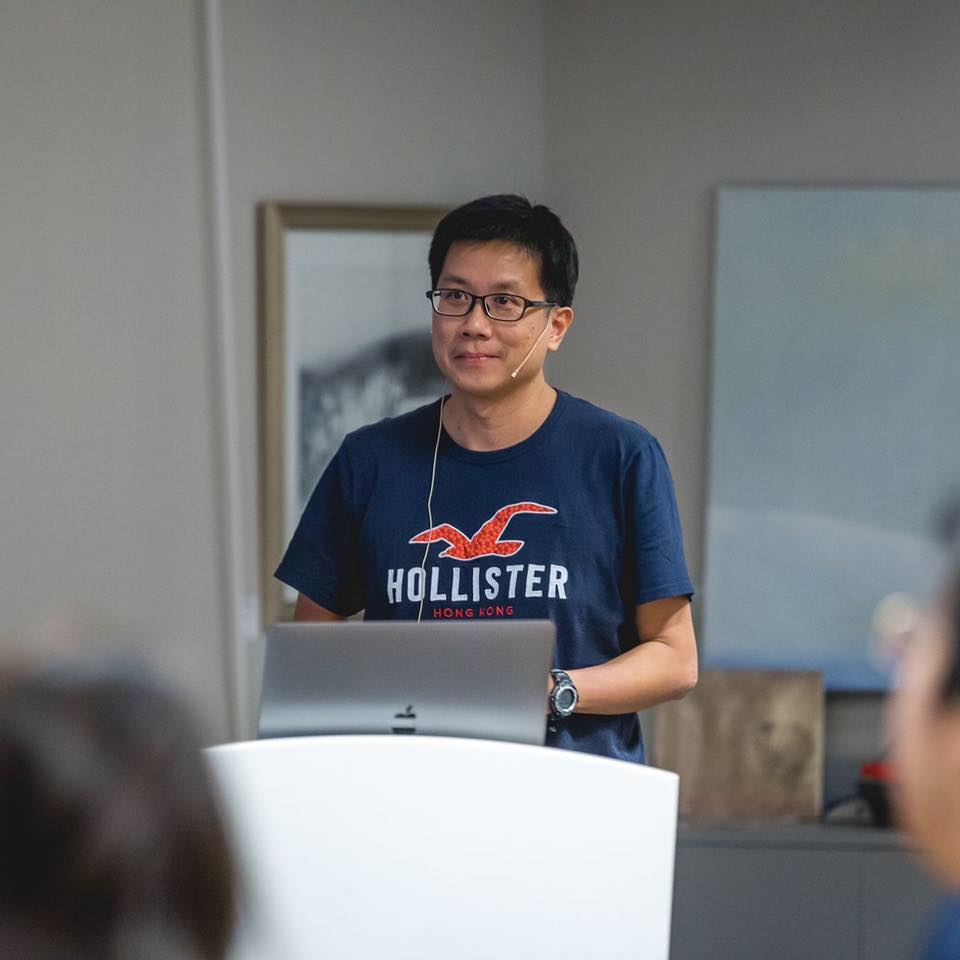
JSON is getting very popular these days. JSON stands for JavaScript Object Notation. It is commonly used in data representation as well as data exchange. Unlike XML, JSON is less verbose, but that is exactly what made it so useful. JSON strings are shorter than its XML equivalent, and parsing JSON strings is a walk in the park. In this post, I will give you a 5-minute walk through of JSON.
JSON Data Types
JSON supports the following data types:
- Object
- String
- Boolean
- Number
- Array
- null
The following sections will elaborate on each of these data types.
Object
An Object is an unordered collection of key:value pairs enclosed in a pair of curly braces ({}
). The following is an example of an empty object:
{}
String
The key in an object must be a String, while the value can be either a String, Boolean, Number, Array, null, or another Object.
The following shows an object with one key:value pair:
{ "firstName": "John" }
An object can have multiple key:value pairs, for example:
{ "firstName": "John", "lastName": "Doe" }
Note the comma (,
) after John and there is no comma after Doe.
Each key in the Object must be unique. For example, the following example is not a valid JSON string:
{ "firstName": "John", "firstName": "Doe" }
Boolean
A Boolean value can either be true or false:
{ "firstName": "John", "lastName": "Doe", "isMember": true }
Number
A Number value can either be an integer, or a floating-point number:
{ "firstName": "John", "lastName": "Doe", "isMember": true, "weight": 79.5, "height": 1.73, "children": 3 }
Nested Object
The value of a key can also be another Object, as the following example shows:
{ "firstName": "John", "lastName": "Doe", "isMember": true, "weight": 79.5, "height": 1.73, "children": 3, "address": { "line1": "123 Street", "line2": "San Francisco", "state": "CA", "postal": "12345" } }
Array
An Array is an ordered sequence of Objects:
{ "firstName": "John", "lastName": "Doe", "isMember": true, "weight": 79.5, "height": 1.73, "children": 3, "address": { "line1": "123 Street", "line2": "San Francisco", "state": "CA", "postal": "12345" }, "phone": [ { "type": "work", "number": "1234567" }, { "type": "home", "number": "8765432" }, { "type": "mobile", "number": "1234876" } ] }
Note that arrays are denoted with a pair of brackets ([]
).
null
When a key has no value, you can assign a null to it:
{ "firstName": "John", "lastName": "Doe", "isMember": true, "weight": 79.5, "height": 1.73, "children": 3, "address": { "line1": "123 Street", "line2": "San Francisco", "state": "CA", "postal": "12345" }, "phone": [ { "type": "work", "number": "1234567" }, { "type": "home", "number": "8765432" }, { "type": "mobile", "number": "1234876" } ], "oldMembershipNo": null }
That's it! Hopefully you now have a better idea of what JSON is. In the next couple of postings, I am going to show you how to manipulate JSON strings using the various languages, such as JavaScript, Swift, Objective-C, Python, and more!